3.1. Initiate payment - Drop Checkout
Drop flow
Drop flow is the standard flow for Cashfree payment gateway's Android SDK. In this flow, SDK provides a pre-built native Android screen to facilitate a quick integration with our payment gateway. Your customers can fill in the necessary details here and complete the payment.
This mode handles all the business logic and UI Components to make the payment smooth and easy to use. The SDK allows the merchant to customize the UI in terms of color coding, fonts and payment modes shown.
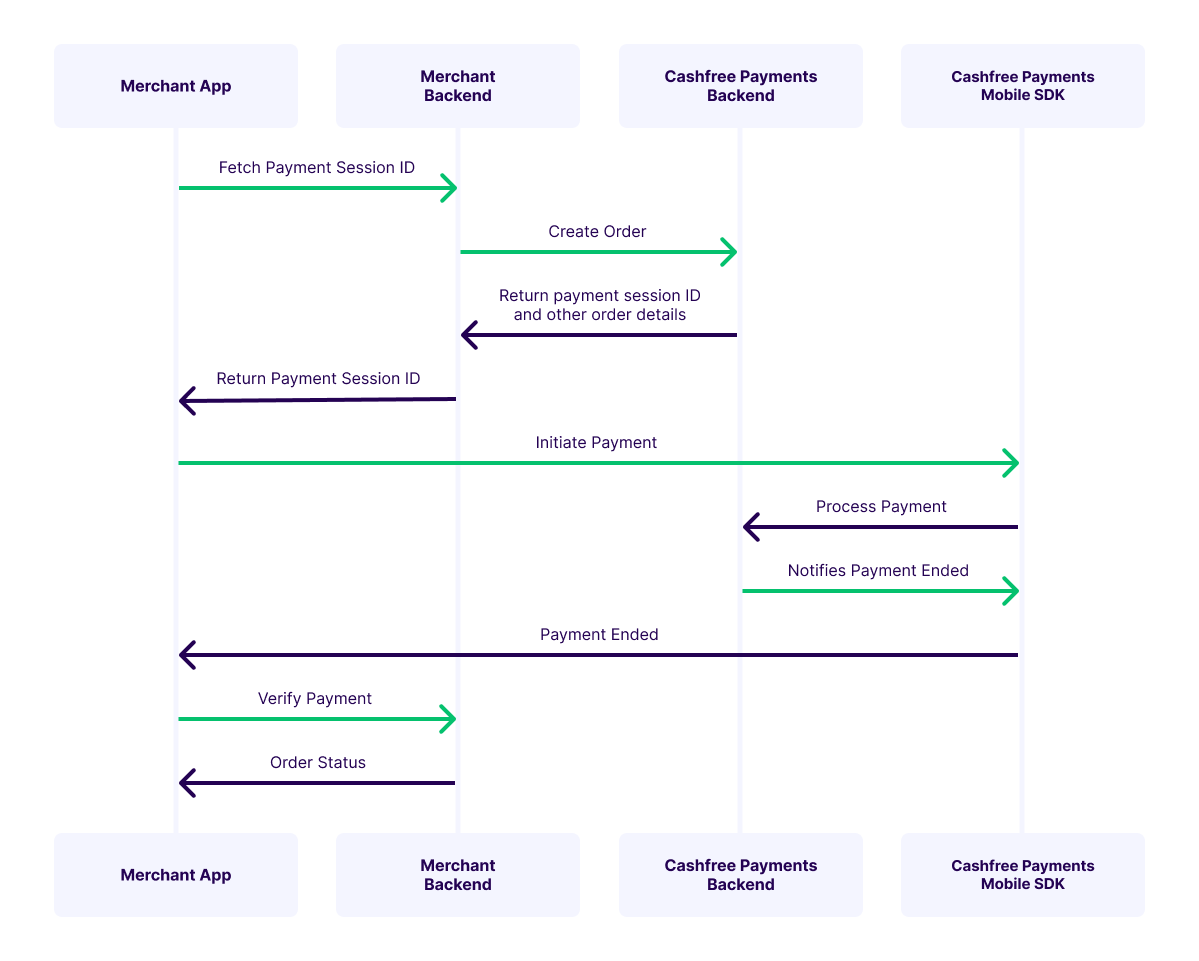
Drop Checkout
Initiating the payment
To initiate the Drop checkout payment in the SDK, follow these steps
- Create a CFSession object.
- Create a CFPaymentComponents object.
- Create a CFTheme object.
- Create a Drop Checkout Payment object.
- Set payment callback.
- Initiate the payment using the payment object created from [step 4]
Create a session
The payment_session_id
created from Step2 is used to authenticate the payment. The SDK exposes a class CFSession
class which accepts the payment_session_id, Environment and order ID values.
Cashfree provides two environments, one being the sandbox
environment for developers to test the payment flow and responses and the other being production
environment which gets shipped to production. This environment can be set in this session object.
The values for environment can be either .SANDBOX
or .PRODUCTION
.
CFSession cfSession = new CFSession.CFSessionBuilder()
.setEnvironment(CFSession.Environment.SANDBOX)
.setPaymentSessionID("paymentSessionID")
.setOrderId("orderID")
.build();
val cfSession: CFSession = CFSessionBuilder()
.setEnvironment(CFSession.Environment.SANDBOX)
.setPaymentSessionID("paymentSessionID")
.setOrderId("orderID")
.build();
Payment Components
The Cashfree's Drop checkout allows the merchant to control the payment modes shown to their customer using the payment component parameter.
CFPaymentComponent cfPaymentComponent = new CFPaymentComponent.CFPaymentComponentBuilder()
.add(CFPaymentComponent.CFPaymentModes.CARD)
.add(CFPaymentComponent.CFPaymentModes.UPI)
.build();
val cfPaymentComponent = CFPaymentComponentBuilder()
.add(CFPaymentComponent.CFPaymentModes.CARD)
.add(CFPaymentComponent.CFPaymentModes.UPI)
.build()
If this method is not called, by default all the payment modes are enabled.
Set a Theme
CFTheme cfTheme = new CFTheme.CFThemeBuilder()
.setNavigationBarBackgroundColor("#6A3FD3")
.setNavigationBarTextColor("#FFFFFF")
.setButtonBackgroundColor("#6A3FD3")
.setButtonTextColor("#FFFFFF")
.setPrimaryTextColor("#000000")
.setSecondaryTextColor("#000000")
.build();
val cfTheme = CFThemeBuilder()
.setNavigationBarBackgroundColor("#006EE1")
.setNavigationBarTextColor("#ffffff")
.setButtonBackgroundColor("#006EE1")
.setButtonTextColor("#ffffff")
.setPrimaryTextColor("#000000")
.setSecondaryTextColor("#000000")
.build()
The CFThemeBuilder
class used to create CFTheme
used to set the theming for Drop checkout screen.
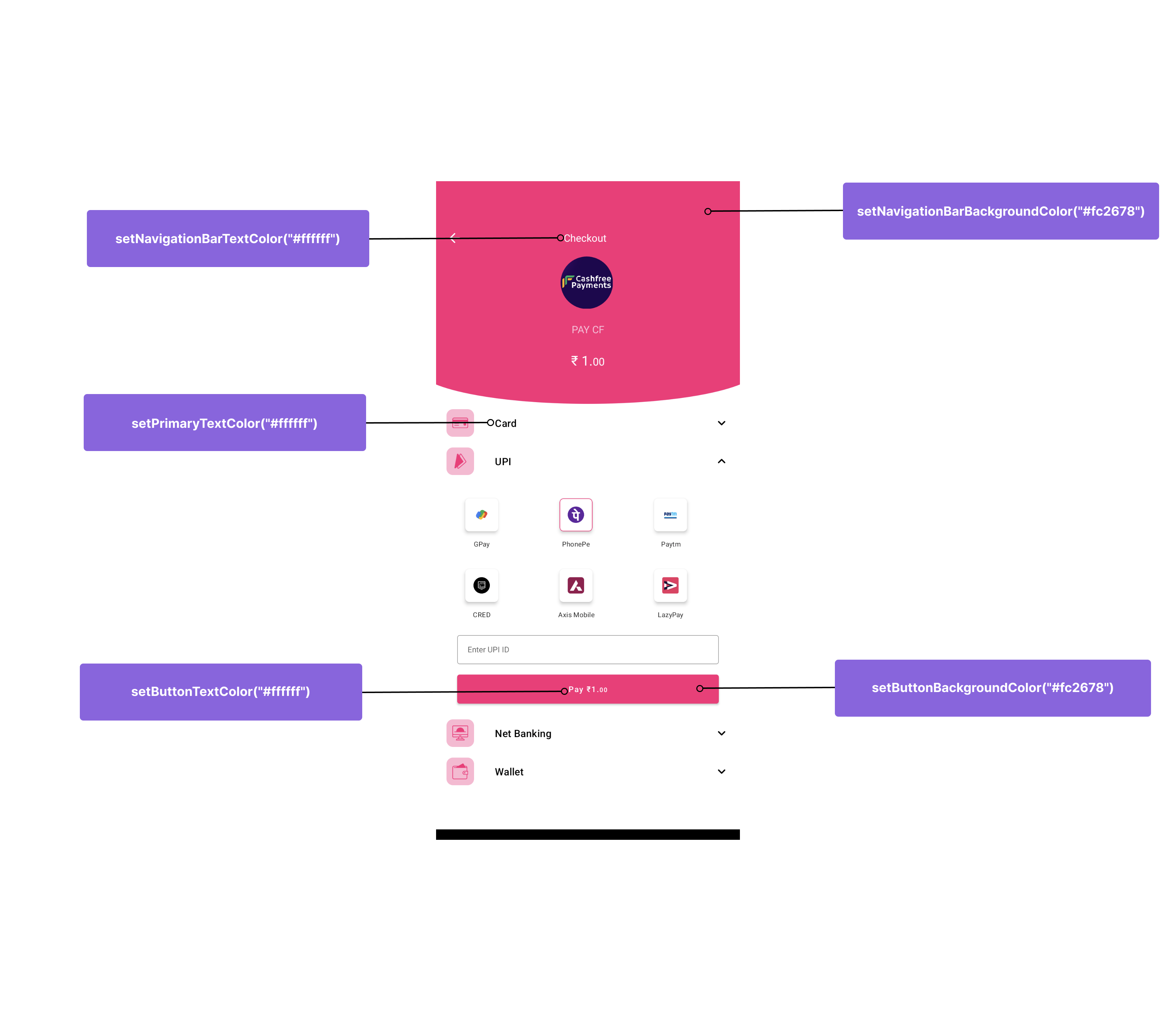
Theme options
Create a Drop Checkout Payment object
- Code Snippet to create a payment object for Drop Checkout (pre-built UI SDK)
CFDropCheckoutPayment cfDropCheckoutPayment = new CFDropCheckoutPayment.CFDropCheckoutPaymentBuilder()
.setSession(cfSession)
.setCFUIPaymentModes(cfPaymentComponent)
.setCFNativeCheckoutUITheme(cfTheme)
.build();
val cfDropCheckoutPayment = CFDropCheckoutPaymentBuilder()
.setSession(cfSession)
.setCFUIPaymentModes(cfPaymentComponent)
.setCFNativeCheckoutUITheme(cfTheme)
.build()
If payment component object is not provided then all the payment modes that are enabled for the merchant account is shown.
Setup Payment Callback
The SDK exposes an interface CFCheckoutResponseCallback
to receive callbacks from the SDK once the payment flow ends.
This protocol comprises of 2 methods:
public void onPaymentVerify(String orderID)
public void onPaymentFailure(CFErrorResponse cfErrorResponse, String orderID)
- Code snippet demonstrating it's usage:
public class YourActivity extends AppCompatActivity implements CFCheckoutResponseCallback {
...
@Override
public void onPaymentVerify(String orderID) {
}
@Override
public void onPaymentFailure(CFErrorResponse cfErrorResponse, String orderID) {
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_drop_checkout);
try {
// If you are using a fragment then you need to add this line inside onCreate() of your Fragment
CFPaymentGatewayService.getInstance().setCheckoutCallback(this);
} catch (CFException e) {
e.printStackTrace();
}
}
...
}
class DropCheckoutActivity : AppCompatActivity(), CFCheckoutResponseCallback {
...
override fun onPaymentVerify(orderID: String) {
}
override fun onPaymentFailure(cfErrorResponse: CFErrorResponse, orderID: String) {
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_drop_checkout)
try {
CFPaymentGatewayService.getInstance().setCheckoutCallback(this)
} catch (e: CFException) {
e.printStackTrace()
}
}
...
}
Make sure to set the callback at activity's onCreate as this also handles the activity restart cases.
Sample Code
package com.cashfree.sdk_sample;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import androidx.appcompat.app.AppCompatActivity;
import com.cashfree.pg.api.CFPaymentGatewayService;
import com.cashfree.pg.core.api.CFSession;
import com.cashfree.pg.core.api.CFTheme;
import com.cashfree.pg.core.api.callback.CFCheckoutResponseCallback;
import com.cashfree.pg.core.api.exception.CFException;
import com.cashfree.pg.core.api.utils.CFErrorResponse;
import com.cashfree.pg.ui.api.CFDropCheckoutPayment;
import com.cashfree.pg.ui.api.CFPaymentComponent;
import com.cashfree.pg.ui.api.base.CFDropCheckoutResponseCallback;
public class DropCheckoutActivity extends AppCompatActivity implements CFCheckoutResponseCallback {
String orderID = "ORDER_ID";
String paymentSessionID = "TOKEN";
CFSession.Environment cfEnvironment = CFSession.Environment.PRODUCTION;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_drop_checkout);
try {
CFPaymentGatewayService.getInstance().setCheckoutCallback(this);
} catch (CFException e) {
e.printStackTrace();
}
}
@Override
public void onPaymentVerify(String orderID) {
Log.e("onPaymentVerify", "verifyPayment triggered");
// Start verifying your payment
}
@Override
public void onPaymentFailure(CFErrorResponse cfErrorResponse, String orderID) {
Log.e("onPaymentFailure " + orderID, cfErrorResponse.getMessage());
}
public void doDropCheckoutPayment() {
try {
CFSession cfSession = new CFSession.CFSessionBuilder()
.setEnvironment(cfEnvironment)
.setPaymentSessionID(paymentSessionID)
.setOrderId(orderID)
.build();
CFPaymentComponent cfPaymentComponent = new CFPaymentComponent.CFPaymentComponentBuilder()
// Shows only Card and UPI modes
.add(CFPaymentComponent.CFPaymentModes.CARD)
.add(CFPaymentComponent.CFPaymentModes.UPI)
.build();
// Replace with your application's theme colors
CFTheme cfTheme = new CFTheme.CFThemeBuilder()
.setNavigationBarBackgroundColor("#fc2678")
.setNavigationBarTextColor("#ffffff")
.setButtonBackgroundColor("#fc2678")
.setButtonTextColor("#ffffff")
.setPrimaryTextColor("#000000")
.setSecondaryTextColor("#000000")
.build();
CFDropCheckoutPayment cfDropCheckoutPayment = new CFDropCheckoutPayment.CFDropCheckoutPaymentBuilder()
.setSession(cfSession)
.setCFUIPaymentModes(cfPaymentComponent)
.setCFNativeCheckoutUITheme(cfTheme)
.build();
CFPaymentGatewayService gatewayService = CFPaymentGatewayService.getInstance();
gatewayService.doPayment(DropCheckoutActivity.this, cfDropCheckoutPayment);
} catch (CFException exception) {
exception.printStackTrace();
}
}
}
package com.cashfree.sdk_sample.kotlin
import androidx.appcompat.app.AppCompatActivity
import com.cashfree.pg.core.api.callback.CFCheckoutResponseCallback
import com.cashfree.pg.core.api.CFSession
import android.os.Bundle
import com.cashfree.sdk_sample.R
import com.cashfree.pg.api.CFPaymentGatewayService
import android.content.Intent
import com.cashfree.pg.core.api.utils.CFErrorResponse
import android.text.TextUtils
import android.util.Log
import android.widget.Toast
import com.cashfree.pg.core.api.CFSession.CFSessionBuilder
import com.cashfree.pg.core.api.CFTheme.CFThemeBuilder
import com.cashfree.pg.core.api.exception.CFException
import com.cashfree.pg.ui.api.CFDropCheckoutPayment.CFDropCheckoutPaymentBuilder
import com.cashfree.sdk_sample.Config
class DropCheckoutActivity : AppCompatActivity(), CFCheckoutResponseCallback {
private val config = Config()
private val orderID = config.orderID
private val paymentSessionID = config.paymentSessionID
private var cfEnvironment = config.environment
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_drop_checkout)
try {
CFPaymentGatewayService.getInstance().setCheckoutCallback(this)
doDropCheckoutPayment()
} catch (e: CFException) {
e.printStackTrace()
}
}
override fun onPaymentVerify(orderID: String) {
Log.d("onPaymentVerify", "verifyPayment triggered")
}
override fun onPaymentFailure(cfErrorResponse: CFErrorResponse, orderID: String) {
Log.e("onPaymentFailure $orderID", cfErrorResponse.message)
}
private fun doDropCheckoutPayment() {
Log.d([email protected], config.toString());
if (orderID == "ORDER_ID" || TextUtils.isEmpty(orderID)) {
Toast.makeText(
this,
"Please set the orderId (DropCheckoutActivity.class, line: 22)",
Toast.LENGTH_SHORT
).show()
finish()
return
}
if (paymentSessionID == "TOKEN" || TextUtils.isEmpty(paymentSessionID)) {
Toast.makeText(
this,
"Please set the token (DropCheckoutActivity.class, line: 23)",
Toast.LENGTH_SHORT
).show()
finish()
return
}
try {
val cfSession: CFSession = CFSessionBuilder()
.setEnvironment(cfEnvironment)
.setPaymentSessionID(paymentSessionID)
.setOrderId(orderID)
.build()
// val cfPaymentComponent = CFPaymentComponentBuilder()
// .add(CFPaymentComponent.CFPaymentModes.CARD)
// .add(CFPaymentComponent.CFPaymentModes.UPI)
// .build()
val cfTheme = CFThemeBuilder()
.setNavigationBarBackgroundColor("#006EE1")
.setNavigationBarTextColor("#ffffff")
.setButtonBackgroundColor("#006EE1")
.setButtonTextColor("#ffffff")
.setPrimaryTextColor("#000000")
.setSecondaryTextColor("#000000")
.build()
val cfDropCheckoutPayment = CFDropCheckoutPaymentBuilder()
.setSession(cfSession) //By default all modes are enabled. If you want to restrict the payment modes uncomment the next line
// .setCFUIPaymentModes(cfPaymentComponent)
.setCFNativeCheckoutUITheme(cfTheme)
.build()
val gatewayService = CFPaymentGatewayService.getInstance()
gatewayService.doPayment(this@DropCheckoutActivity, cfDropCheckoutPayment)
} catch (exception: CFException) {
exception.printStackTrace()
}
}
}
Verify Payment
As a best practise you should always verify the payment status from your backend. Refer here for details steps on how to verify payment.
Updated 8 months ago