Step 3: Initiate Payment - Web checkout payment
Web Checkout flow
Web checkout flow is a payment flow for collection payments using the Cashfree payment gateway's iOS SDK. In this flow, SDK provides a webview based checkout implementation to facilitate a quick integration with our payment gateway. Your customers can fill in the necessary details in the web page and complete the payment.
This mode also handles all the business logic and UI Components to make the payment smooth and easy to use.
We have added this mode for giving access to some features which are only available in the web checkout flow now.
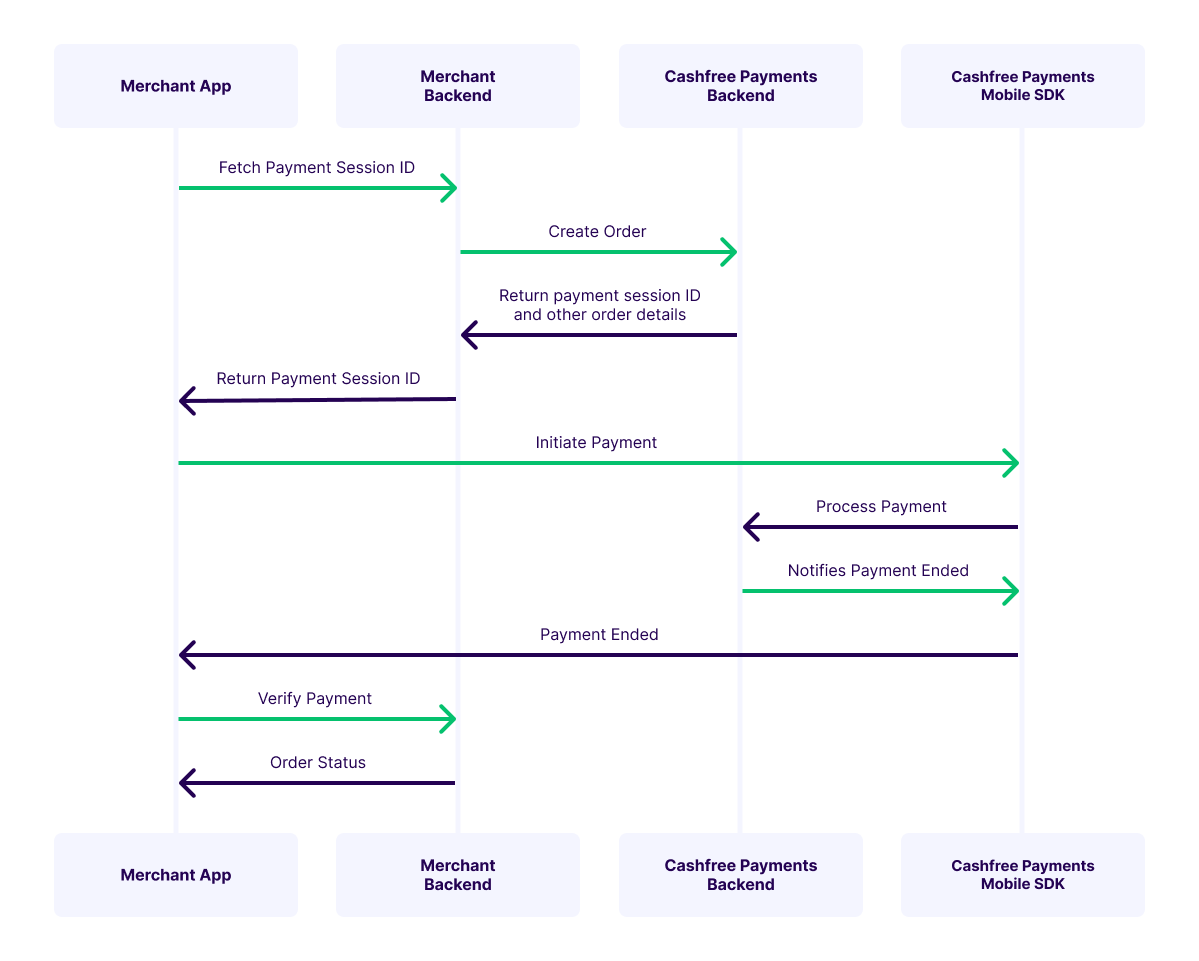
Web Checkout
Initiating the payment
To initiate the Web checkout payment in the SDK, follow these steps
- Create a CFSession object.
- Create a Web Checkout Payment object.
- Set payment callback.
- Initiate the payment using the payment object created.
Create a session
The payment_session_id
created from Step2 is used to authenticate the payment. The SDK exposes a class CFSession
class which accepts the payment_session_id, Environment and order ID values.
Cashfree provides two environments, one being the sandbox
environment for developers to test the payment flow and responses and the other being production
environment which gets shipped to production. This environment can be set in this session object.
The values for environment can be either .SANDBOX
or .PRODUCTION
.
do {
let session = try CFSession.CFSessionBuilder()
.setOrderID(order_id)
.setPaymentSessionId(payment_session_id)
.setEnvironment(Utils.environment)
.build()
return session;
} catch let e {
let error = e as! CashfreeError
self.createAlert(title: "Warning", message: error.localizedDescription)
}
@try {
CFSessionBuilder* sessionBuilder = [[CFSessionBuilder alloc] init];
sessionBuilder = [sessionBuilder setPaymentSessionId:paymentSessionId];
sessionBuilder = [sessionBuilder setOrderID:orderId];
sessionBuilder = [sessionBuilder setEnvironment:CFENVIRONMENTPRODUCTION];
CFSession* session = [sessionBuilder buildAndReturnError:nil];
} @catch (NSException *exception) {
NSLog(@"%@", exception);
}
Create a Web Checkout Payment object
- Code Snippet to create a payment object for Web Checkout (pre-built Web UI SDK)
let webCheckoutPayment = try CFWebCheckoutPayment.CFWebCheckoutPaymentBuilder()
.setSession(session)
.build()
@try {
CFWebCheckoutPaymentBuilder* web = [[CFWebCheckoutPaymentBuilder alloc] init];
web = [web setSession:session];
CFWebCheckoutPayment* webPayment = [web buildAndReturnError:nil];
} @catch (NSException *exception) {
NSLog(@"%@", exception);
}
Setup Payment Callback
extension ViewController: CFResponseDelegate {
func onError(_ error: CFErrorResponse, order_id: String) {
self.createAlert(title: error.status ?? "ERROR", message: error.message ?? "error_message_not_present")
}
func verifyPayment(order_id: String) {
self.createAlert(title: "VERIFY PAYMENT", message: "Payment has to be verified by merchant for \(order_id)")
}
}
// Class Variable
let pgService = CFPaymentGatewayService.getInstance()
override func viewDidLoad() {
super.viewDidLoad()
pgService.setCallback(self)
}
Sample Code
import CashfreeAnalyticsSDK
import CashfreePG
import CashfreePGCoreSDK
import CashfreePGUISDK
class ViewController: UIViewController, CFResponseDelegate {
// Class Variable
let pgService = CFPaymentGatewayService.getInstance()
override func viewDidLoad() {
super.viewDidLoad()
pgService.setCallback(self)
}
@IBAction func webCheckoutButtonTapped(_ sender: Any) {
do {
let session = try CFSession.CFSessionBuilder()
.setPaymentSessionId(payment_session_id)
.setOrderID(order_id)
.setEnvironment(Utils.environment)
.build()
let webCheckoutPayment = try CFWebCheckoutPayment.CFWebCheckoutPaymentBuilder()
.setSession(session)
.build()
try pgService.doPayment(webCheckoutPayment, viewController: self)
} catch let e {
let err = e as! CashfreeError
print(err.description)
}
}
// Protocol Implementation
func onError(_ error: CFErrorResponse, order_id: String) {
self.createAlert(
title: error.status ?? "ERROR", message: error.message ?? "error_message_not_present")
}
func verifyPayment(order_id: String) {
self.createAlert(
title: "VERIFY PAYMENT", message: "Payment has to be verified by merchant for \(order_id)")
}
}
@try {
CFSessionBuilder* sessionBuilder = [[CFSessionBuilder alloc] init];
sessionBuilder = [sessionBuilder setPaymentSessionId:paymentSessionId];
sessionBuilder = [sessionBuilder setOrderID:orderId];
sessionBuilder = [sessionBuilder setEnvironment:CFENVIRONMENTPRODUCTION];
CFSession* session = [sessionBuilder buildAndReturnError:nil];
CFWebCheckoutPaymentBuilder* web = [[CFWebCheckoutPaymentBuilder alloc] init];
web = [web setSession:session];
CFWebCheckoutPayment* webPayment = [web buildAndReturnError:nil];
CFPaymentGatewayService* pg = [CFPaymentGatewayService alloc];
[pg setCallback:self];
[pg doPayment:webPayment viewController:self error:nil];
} @catch (NSException *exception) {
NSLog(@"%@", exception);
}
Verify Payment
As a best practise you should always verify the payment status from your backend. Refer here for details steps on how to verify payment.
Subscribe to Developer Updates
Updated 6 months ago