Getting Started
Initialize with script tag
Include the cashfree.js
, make sure that it is loaded directly from https://sdk.cashfree.com
. To be PCI compliant, you must load cashfree.js directly from https://sdk.cashfree.com. You cannot include it in a bundle or host it yourself.
<script src="https://sdk.cashfree.com/js/v3/cashfree.js"></script>
How to always use a specific version
To always use a specific version you will have to include the version in this way
<script src="https://sdk.cashfree.com/js/v3/cashfree-2023.03.07.js"></script>
We recommend to use the latest version (cashfree.js) always. You can find all the versions here
Asynchronous and deferred loading of Cashfree.js
Asynchronous loading of JavaScript is generally recommended, as it can improve the user experience of your site by not blocking DOM rendering during script loading.
You can also load cashfree.js using the async
or defer
attribute on the script tag. Kindly note that with asynchronous loading, any API calls will have to be made only after the script execution has finished.
To initialize
const cashfree = Cashfree({
mode:"sandbox" //or production
});
mode
typestring
REQUIRED
It can be either sandbox
or production
depending upon the URL you used for creating payment_session_id
-
-
https://api.cashfree.com/pg/orders
- then it will beproduction
-
https://sandbox.cashfree.com/pg/orders
- then it will besandbox
Returns
An instance of Cashfree
INTEGRATION TOOLKIT
Try our Integration Try the API's using PostmanInitialize as a module
Installation
npm install @cashfreepayments/cashfree-js
Initialize
import {load} from '@cashfreepayments/cashfree-js';
const cashfree = await load({
mode: "sandbox" //or production
});
This load
function returns a Promise that resolves with a newly created Cashfree object once Cashfree.js has loaded. If you call load in a server environment it will resolve to null.
If you have deployed a Content Security Policy, make sure to Cashfree domains in your directives.
connect-src
,https://api.cashfree.com
frame-src
,https://sdk.cashfree.com
,https://api.cashfree.com
,https://sandbox.cashfree.com
https://payments.cashfree.com
,https://payments-test.cashfree.com
script-src
,https://sdk.cashfree.com
Methods of cashfree
variable
cashfree
variableBelow are few methods that you can call on the above variable
version()
Get the version of the sdk
How to use
let version = cashfree.version();
Returns
A string, which references the current version that the sdk is using. This will help you understand which version of cashfree.js
your site is using. This is helpful in understanding features available for a version. By default we will always upgrade your sdk to the latest version. But in some cases your customer's browser might cache the javascript for some amount of time. In that case your customers will not get the latest version. Checkout all versions here
checkout()
The checkout method redirects the user to a Cashfree hosted page. See all the supported options here. You can find a full example here
How to use
let checkoutOptions = {
paymentSessionId: "payment-session-id",
returnUrl: "https://test.cashfree.com/pgappsdemos/v3success.php?myorder={order_id}",
}
cashfree.checkout(checkoutOptions).then(function(result){
if(result.error){
alert(result.error.message)
}
if(result.redirect){
console.log("Redirection")
}
});
Returns
cashfree.checkout
will return a Promise
that resolves with either result.error
when there is an error or result.redirect
when there is redirection
create()
Use create()
to initialize a cashfree component for your HTML page.
How to use
Here is a small example demonstrating how to render a UPI collect component. We will see more detailed example in the UPI collect example
Refer to this page for all available components
let options = {
values: {
upiId: "rohit@icici"
}
};
let component = cashfree.create("upiCollect", options);
create
takes two arguments. The first argument is the component name of type string
. You can find all the names of the components we provide here.
The second argument is of type object
. It contains details about the behavior and look of the component. Find all the details for options
object here
Returns
An instance of the component. A component has several methods that you can find here
mount()
If you are building your own checkout page Cashfree provides a set of components. A component can be mounted in DOM container. Use mount()
to mount the component.
How to use
Syntax: component.mount(querySelector)
Example
//let's create a div which will have our component
const div = document.createElement('div');
div.setAttribute("id", "componentContainer");
document.body.appendChild(div);
component.mount("#componentContainer")
pay()
Use pay()
to begin payment flow for a component. Not all components are payable. Check all the components here. The pay method takes in a paymentOption object.
How to use
let paymentOptions = {
paymentMethod: component,
paymentSessionId: "your-payment-session-id",
returnUrl: "https://example.com?order={order_id}"
}
cashfree.pay(paymentOptions).then(function (result) {
if(result.error) {
//there is an error
//message at result.error.message
}
if(result.redirect){
//console.log("User would be redirected");
}
if(result.paymentDetails) {
//only when redirect = 'if_required' for UPI payment
//payment is successful
//message is at result.paymentDetails.paymentMessage
}
});
You can read more about payment options and their configuration here
Returns
cashfree.pay
will return a Promise
.
Upon success, the promise will resolve with result.paymentMessage
if the user has opted for a non redirection flow. example UPI, QR.
Upon redirection, the promise will resolve with result.redirect
If the payment fails, the Promise
will resolve with an result.error
. error.type
will describe the failure. In some cases you can directly show the error.message to your user. Please refer here for the types
Note that cashfree.pay
may take several seconds to complete. During that time, you should disable user interaction and show a waiting indicator like a spinner. If you receive an error result, you should be sure to show that error to the customer, re-enable user interaction, and hide the waiting indicator.
What to do after payment is complete
After payment have been completed by your customer, you would want to destroy all your payment components or redirect your customer to a order confirmation page. This has to be built by you.
Component Lifecycle
A component lifecycle starts with create and it ends when payment is completed for the component or component is manually destroyed. We will take example of upiCollect component here
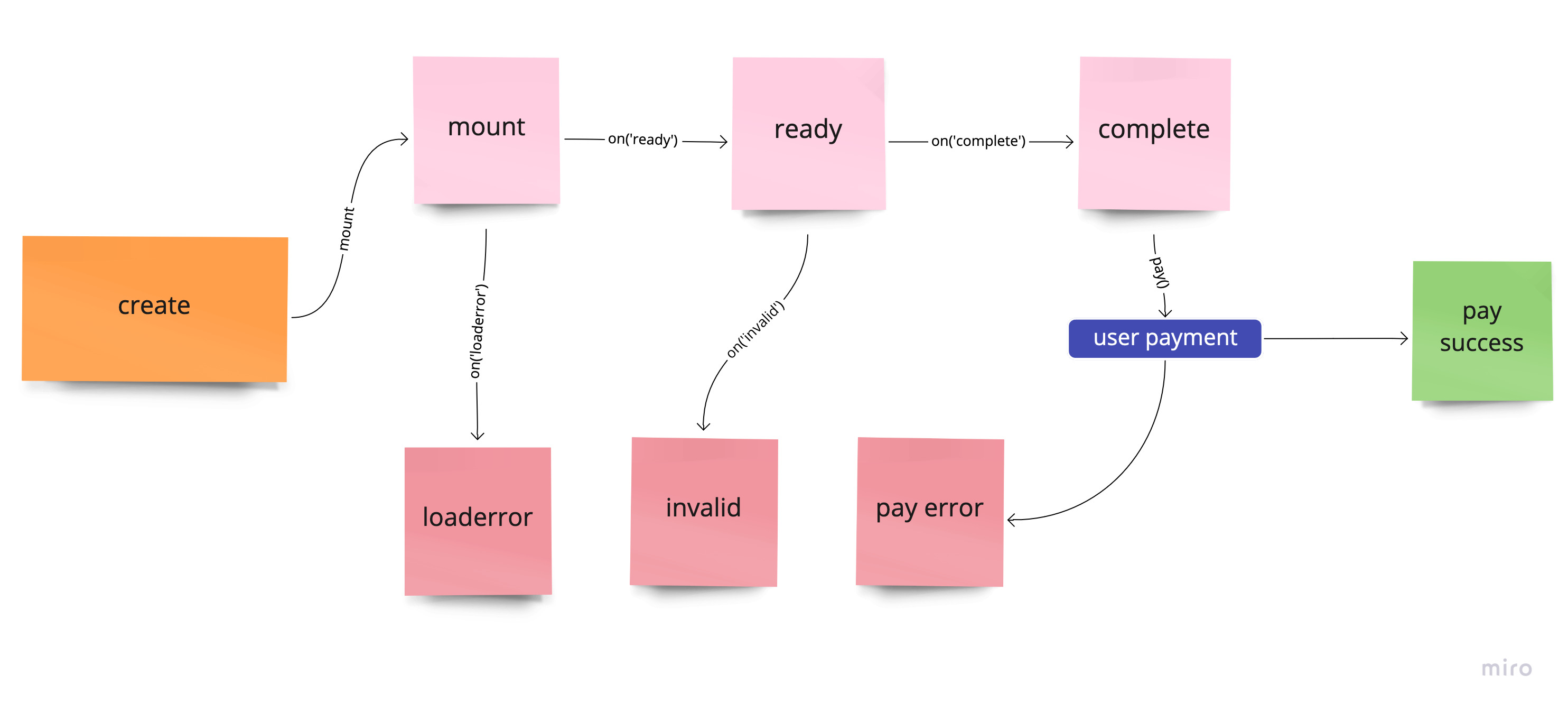
component lifecycle
- Include cashfree in your HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="my-div"></div>
<button id="pay">Pay</button>
<script src="https://sdk.cashfree.com/js/v3/cashfree.js"></script>
</body>
</html>
- Init cashfree using cashfree.create()
const cashfree = Cashfree({
mode: "sandbox",
});
- Create component
let options = {
values:{
upiId: "testsuccess@gocash"
}
};
let component = cashfree.create("upiCollect", options);
Read about different values that options can take here
- Mount component to DOM. There are multiple component methods. You can find the complete list here
component.mount("#my-div");
- Initiate Payment. Get a sample
payment_session_id
here
document.getElementById("pay").addEventListener("click", function(){
let paymentPromise = cashfree.pay({
paymentMethod: component,
paymentSessionId: "payment_session_id",
returnUrl: "https://test.cashfree.com/pgappsdemos/v3success.php?myorder={order_id}"
});
paymentPromise.then(function(result){
console.log(result);
})
})
Subscribe to Developer Updates
Updated 6 months ago