Standard Transfer
This section provides details on how to integrate a standard transfer. It explains how to add a beneficiary, make a payment, and get the transfer status.
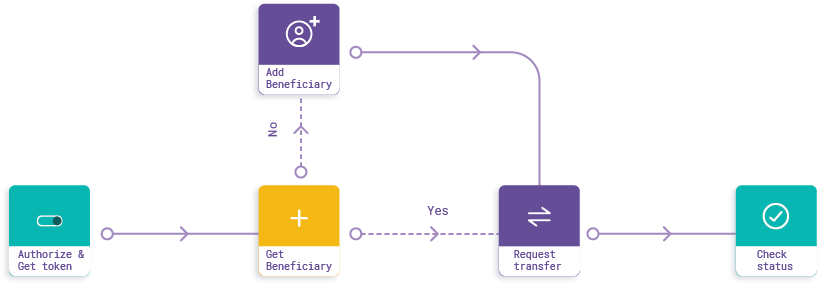
Standard Transfer
Steps
- Setup
- Initialization and Authorization
- Get beneficiary details
- Add a beneficiary
- Request a transfer
- Get transfer status
Check out our libraries and samples section for the integration code hosted on GitHub.
Step 1: Setup
Get your corresponding clientId and clientSecret from your payout dashboard and ensure that your IP is whitelisted as well. Check our development quickstart here.
Host URL: Use the following URL for PROD and TEST, respectively:
- For the production environment: https://payout-api.cashfree.com
- For the test environment: https://payout-gamma.cashfree.com
Step 2: Initialization and Authorization
Call the authenticate API to Cashfree system/server to obtain an Authorization Bearer token. All other API calls must have this token as Authorization header in the format 'Bearer ' (without quotes) to get processed.
curl -X POST \
'https://payout-api.cashfree.com/payout/v1/authorize' \
-H 'X-Client-Id: {{client id}}' \
-H 'X-Client-Secret: {{client secret}}' \
-H 'cache-control: no-cache'
//require CashfreeSDK
const cfSdk = require('cashfree-sdk');
//access the PayoutsSdk from CashfreeSDK
const {Payouts} = cfSdk;
// Instantiate Cashfree Payouts
const payoutsInstance = new Payouts({
env: 'TEST',
clientId: '<CLIENT_ID>',
clientSecret: '<CLIENT_SECRET>',
});
from cashfree_sdk.payouts import Payouts
from cashfree_sdk.payouts.beneficiary import Beneficiary
from cashfree_sdk.payouts.transfers import Transfers
clientId = "your_clientId"
clientSecret = "your_client_secret"
env = "TEST"
Payouts.init(clientId, clientSecret, env)
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.constants.Constants.Environment;
public static void main() {
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
}
Sample response
// This is a curl response
{
"status":"SUCCESS",
"message":"Token generated",
"subCode":"200",
"data": {"token":"eyJ0eXA...fWStg", "expiry":1564130052}
}
Step 3: Get Beneficiary Details
Fetch the details of a beneficiary by passing the beneficiary Id. beneId is a unique identifier for your beneficiary.
curl -X GET \
'https://payout-api.cashfree.com/payout/v1/getBeneficiary/JOHN18011343' \
-H 'Authorization: Bearer {{Token}}' \
-H 'Postman-Token: 59c129af-c109-482a-bb6f-6fc18abde21d' \
-H 'cache-control: no-cache'
//fetch beneficiary details.
const response = await payoutsInstance.beneficiary.getById({
"beneId": "JOHN18011343",
});
#fetch beneficary details
bene_details_response = Beneficiary.get_bene_details("JOHN18011343")
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Beneficiary;
import com.cashfree.lib.domains.BeneficiaryDetails;
public static void main(){
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
Beneficiary beneficiary = new Beneficiary(payouts);
beneficiary.getBeneficiaryDetails("JOHN18011343");
}
Sample Response
{
"status":"SUCCESS",
"subCode":"200",
"message":"Details of beneficiary",
"data":
{
"beneId":"JOHN18011343",
"name":"John Doe","groupName":"DEFAULT",
"email":"[email protected]",
"phone":"9876543210",
"address1":"ABC street",
"address2":"",
"city":"Bangalore",
"state":"Karnataka",
"pincode":"0",
"bankAccount":"00011020001772",
"ifsc":"HDFC0000001",
"status":"VERIFIED"
}
}
Step 4: Add Beneficiary
Add a beneficiary by providing the bank account number, IFSC, and other required details. You can only request a transfer for added beneficiaries.
curl -X POST \
'https://payout-api.cashfree.com/payout/v1/addBeneficiary' \
-H 'Authorization: Bearer {{Token}}' \
-d '{
"beneId": "JOHN18011343",
"name": "john doe",
"email": "[email protected]",
"phone": "9876543210",
"bankAccount": "00111122233",
"ifsc": "HDFC0000001",
"address1": "ABC Street",
"city": "Bangalore",
"state": "Karnataka",
"pincode": "560001"
}'
//add beneficiary
const response = await payoutsInstance.beneficiary.add({
"beneId": "JOHN180124",
"name": "john doe",
"email": "[email protected]",
"phone": "9876543210",
"bankAccount": "00011020001772",
"ifsc": "HDFC0000001",
"address1" : "ABC Street",
"city": "Bangalore",
"state":"Karnataka",
"pincode": "560001"
});
from cashfree_sdk.payouts.beneficiary import Beneficiary
bene_add_response = Benefeciary.add(
beneId = "JOHN180124"
name = "john doe",
email = "[email protected]",
phone = "9876543213",
bankAccount = "00011020001772",
ifsc = "HDFC0000001",
address1 = "ABC Street",
city = "Bangalore",
stat = "Karnataka",
pincode = "560001"
})
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Beneficiary;
import com.cashfree.lib.domains.BeneficiaryDetails;
public static void main(){
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
Beneficiary beneficiary = new Beneficiary(payouts);
BeneficiaryDetails beneficiaryDetails = new BeneficiaryDetails()
.setBeneId("JOHN18012")
.setName("john doe")
.setEmail("[email protected]")
.setPhone("9876543210")
.setBankAccount("00001111222233")
.setIfsc("HDFC0000001")
.setAddress1("ABC Street")
.setCity("Bangalore")
.setState("Karnataka")
.setPincode("560001");
beneficiary.addBeneficiary(beneficiaryDetails);
}
Sample Response
{
"status":"SUCCESS",
"subCode":"200",
"message":"Beneficiary added successfully"
}
Step 5: Request Transfer
API to request a payout transfer.
curl -X POST \
'https://payout-api.cashfree.com/payout/v1/requestTransfer' \
-H 'Authorization: Bearer {{Token}}' \
-d '{
"beneId": "JOHN18011343",
"amount": "1.00",
"transferId": "JUNOB2018"
}'
//request transfer
const response = await payoutsInstance.transfers.requestTransfer({
"beneId": "JOHN180124",
"transferId": "tranfer001234",
"amount": "1.00",
});
#request transfer
Transfers.request_transfer(
beneId = "JOHN180124",
transferId = "tranfer001234",
amount = "1.00",
)
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Transfers;
import com.cashfree.lib.domains.request.BatchTransferRequest;
import com.cashfree.lib.domains.request.RequestTransferRequest;
public static void main(){
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
Transfers transfer = new Transfers(payouts);
RequestTransferRequest request = new RequestTransferRequest()
.setBeneId("JOHN18012")
.setAmount(new BigDecimal("1.00"))
.setTransferId("javasdktestJAN2019");
transfers.requestTransfer(request);
}
Sample Response
{
"status":"SUCCESS",
"subCode":"200",
"message":"Transfer completed successfully",
"data":
{
"referenceId":"10023",
"utr":"P16111765023806",
"acknowledged": 1
}
}
Step 6: Get Transfer Status
API to fetch details of a particular transfer. You can either pass referenceId or transferId to fetch the details.
This API gives responses other than SUCCESS and ERROR.
curl -X GET \
'https://payout-api.cashfree.com/payout/v1.1/getTransferStatus?referenceId=14057&transferId=tranfer001234' \
-H 'Authorization: Bearer {{Token}}' \
//get transfer status
const response = await payoutsInstance.transfers.getTransferStatus({
"transferId": "tranfer001234",
});
#get transfer status
get_transfer_status_response = Transfers.get_transfer_status(transferId = "tranfer001234")
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Transfers;
import com.cashfree.lib.domains.request.BatchTransferRequest;
import com.cashfree.lib.domains.request.RequestTransferRequest;
public static void main(){
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
Transfers transfer = new Transfers(payouts);
transfers.getTransferStatus("83345068", null);
}
Sample Response
{
"status": "SUCCESS",
"subCode": "200",
"message": "Details of transfer with transferId tranfer001234",
"data": {"transfer": { "referenceId": 17073,
"bankAccount": "026291800001191",
"beneId": "ABCD_123",
"amount": "20.00",
"status": "SUCCESS",
"utr": "1387420170430008800069857",
"addedOn": "2017-01-07 20:09:59",
"processedOn": "2017-01-07 20:10:05",
"acknowledged": 1
}
}
}
Check out our Libraries and Samples section for the integration code hosted on GitHub.
You now have a complete standard transfer integration for payouts. Cashfree will send webhooks in the case of certain events. Webhooks are server to server notifications. Learn more about webhooks here.
When testing your integration with your test API key, you can use test numbers to ensure that it works correctly.
Updated 5 months ago