Cashgram Integration
Cashgram is a sub-service of payouts offered by Cashfree that enables a merchant to send money to their client instantly. This topic will help you to integrate with Cashgram It includes creating a Cashgram and fetching the Cashgram status.
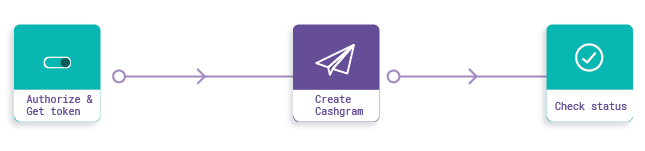
Cashgram Integration Flow
Steps
- Setup
- Initialization and Authorization
- Create Cashgram
- Get Cashgram Status
Step 1: Setup
Get your corresponding clientId and clientSecret from your payout dashboard and ensure that your IP is whitelisted. Check our development quickstart here.
Host URL: Use the following URL for PROD and TEST, respectively:
For the production environment: https://payout-api.cashfree.com
For the test environment: https://payout-gamma.cashfree.com
Step 2: Initialization and Authorization
Call the authenticate API to Cashfree's system/server to obtain an Authorization Bearer token. All other API calls must have this token as Authorization header in the format βBearer β (without quotes) to get processed.
curl -X POST \
'http://{{Host%20Url}}/payout/v1/authorize' \
-H 'X-Client-Id: {{client id}}' \
-H 'X-Client-Secret: {{client secret}}' \
-H 'cache-control: no-cache'
//require CashfreeSDK
const cfSdk = require('cashfree-sdk');
//access the PayoutsSdk from CashfreeSDK
const {Payouts} = cfSdk;
// Instantiate Cashfree Payouts
const payoutsInstance = new Payouts({
env: 'TEST',
clientId: '<CLIENT_ID>',
clientSecret: '<CLIENT_SECRET>',
});
from cashfree_sdk.payouts import Payouts
from cashfree_sdk.payouts.cashgram import Cashgram
clientId = "your_client_id"
clientSecret = "your_client_secret"
env = "TEST"
Payouts.init(clientId, clientSecret, env)
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.constants.Constants.Environment;
public static void main() {
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
}
Sample Response
{
"status":"SUCCESS",
"message":"Token generated",
"subCode":"200",
"data": {"token":"eyJ0eXA...fWStg", "expiry":1564130052}
}
Step 3: Create Cashgram
Create a Cashgram that can be sent directly to your end customer by passing their relevant information.
curl -X POST \
'https://{{Host%20Url}}/payout/v1/payout/v1/createCashgram' \
-H 'Accept: */*' \
-H 'Authorization: Bearer {{token}}' \
-H 'Content-Type: application/json' \
-d '{
"cashgramId": "cf6",
"amount": "1.00",
"name": "sameera",
"email": "[email protected]",
"phone": "9000000001",
"linkExpiry": "2019/11/9",
"remarks": "sample cashgram",
"notifyCustomer": 1
}'
//create cashgram
const response = await payoutsInstance.cashgram.create({
cashgramId: "cf11",
amount: "1.00",
name: "sameera",
email: "[email protected]",
phone: "9000000001",
linkExpiry: "2020/01/12",
remarks: "sample cashgram",
notifyCustomer: 1
});
#create cashgram
create_cashgram_response = Cashgram.create_cashgram(
cashgramId = "cf11",
amount = "1.00",
name = "sameera",
email = "[email protected]",
phone = "9000000001",
linkExpiry = "2020/01/19",
remarks = "sample cashgram",
notifyCustomer = 1
)
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Cashgram;
import com.cashfree.lib.domains.CashgramDetails
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
Cashgram cashgram = new Cashgram(payouts);
CashgramDetails cashgramDetails = new CashgramDetails()
.setCashgramId("javasdk-test2")
.setAmount(new BigDecimal("1.00"))
.setName("John Doe")
.setEmail("[email protected]")
.setPhone("9876543210")
.setLinkExpiry(LocalDateTime.now().plusMinutes(0))
.setRemarks("api")
.setNotifyCustomer(1);
cashgram.createCashgram(cashgramDetails);
}
notifyCustomer field is an optional field when set to 1 it sends the Cashgram to your end customer phone and email.
Sample Response
{
"status": "SUCCESS",
"subCode": "200",
"message": "Cashgram Created",
"data":
{ "referenceId": 3645 ,
"cashgramLink": "http://bit.ly/2xxnGm8"
}
}
Step 4: Get Cashgram Status
Get the status of the created Cashgram by passing the cashgramId.
curl -X GET \
'https://{{Host%20Url}}/payout/v1/getCashgramStatus?cashgramId=JOHaN10' \
-H 'Accept: */*' \
-H 'Authorization: Bearer {{token}}' \
-H 'Content-Type: application/json' \
//get cashgram status
payoutsInstance.cashgram.getStatus({
"cashgramId":cashgram.cashgramId
});
get_cashgram_status_response = Cashgram.get_cashgram_status("cf11")
import com.cashfree.lib.clients.Payouts;
import com.cashfree.lib.clients.Cashgram;
import com.cashfree.lib.domains.CashgramDetails
Payouts payouts = Payouts.getInstance(
Environment.PRODUCTION, "<client_id>", "<client_secret>");
payouts.init();
Cashgram cashgram = new Cashgram(payouts);
cashgram.getCashgramStatus("javasdk-test2");
}
Sample Response
{
"status": "SUCCESS",
"subCode": "200",
"data": { "cashgramStatus": "EXPIRED",
"reason": "TRANSFER_ATTEMPTS_EXCEEDED" }
}
Check out our libraries and samples for the integration code hosted on GitHub.
You now have a complete Cashgram integration for payouts. Cashfree will send webhooks in the case of certain events. Webhooks are events that send you notifications. Learn more about webhooks here.
When testing the integration with your test API key, you can use test numbers to ensure that it works correctly.
Updated 8 months ago