3. Initiate payment - Drop Checkout
Drop flow
Drop flow is the standard flow for Cashfree payment gateway's Cordova SDK. In this flow, SDK provides a pre-built native screen to facilitate a quick integration with our payment gateway. Your customers can fill in the necessary details here and complete the payment.
This mode handles all the business logic and UI Components to make the payment smooth and easy to use. The SDK allows the merchant to customize the UI in terms of color coding and payment modes shown.
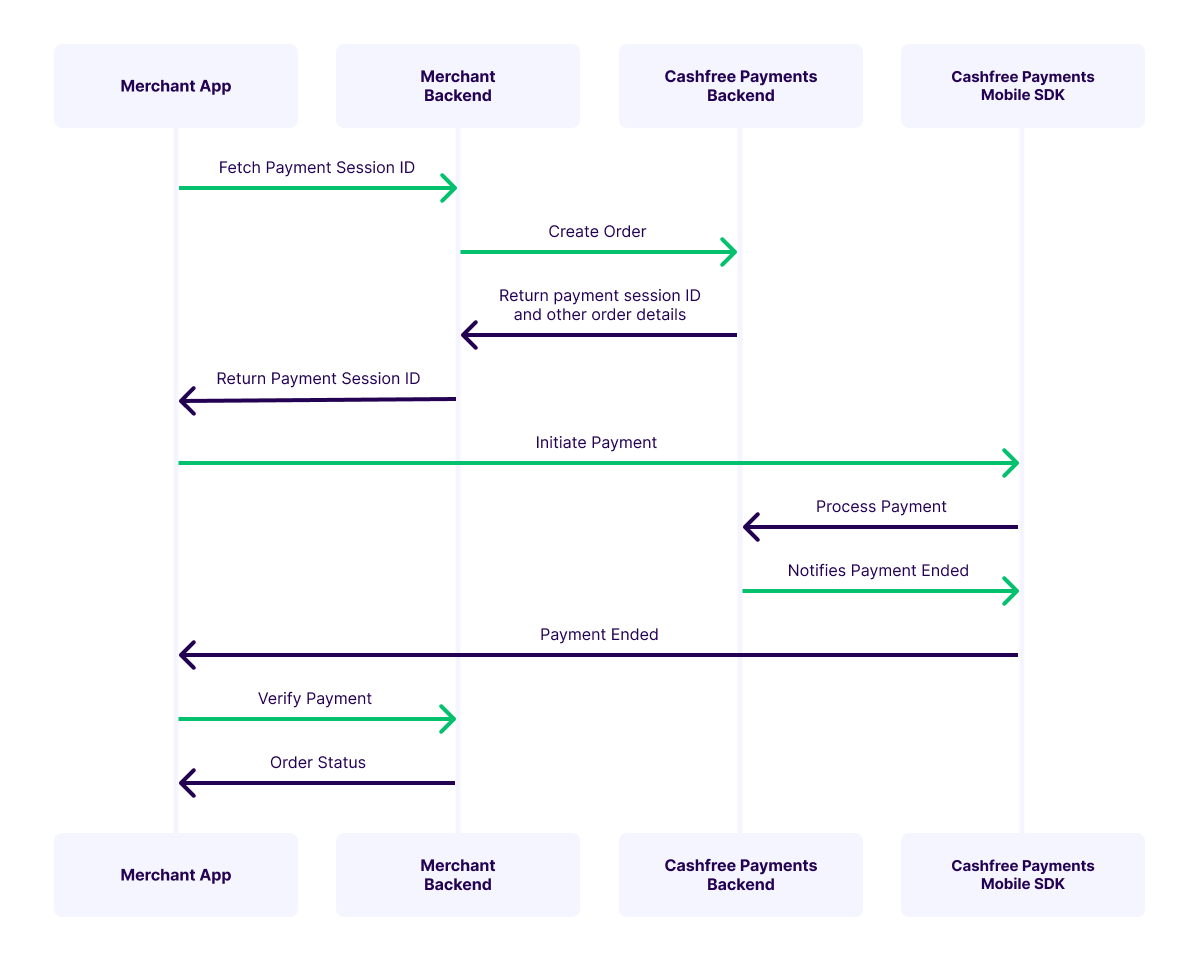
Drop Checkout
Initiating the payment
To initiate the Drop checkout payment in the SDK, follow these steps
- Create a Session object.
- Create a Components object.
- Create a Theme object.
- Create a Drop Checkout Payment object.
- Set payment callback.
- Initiate the payment using the payment object created from [step 4]
Create a session
The payment_session_id
created from Step 2 is used to authenticate the payment.
Cashfree provides two environments, one being the sandbox
environment for developers to test the payment flow and responses and the other being production
environment which gets shipped to production. This environment can be set in this session object.
The values for environment can be either SANDBOX
or PRODUCTION
.
let session = {
"payment_session_id": "payment_session_id",
"orderID": "order_id",
"environment": "SANDBOX" //"SANDBOX" or "PRODUCTION"
}
Payment Components
The Cashfree's Drop checkout allows the merchant to control the payment modes shown to their customer using the payment component parameter.
let components = ["CARD", "UPI", "NB", "WALLET", "PAY_LATER", "EMI"]
If this method is not called, by default all the payment modes are enabled.
Set a Theme
let theme = {
"navigationBarBackgroundColor": "#E64A19",
"navigationBarTextColor": "#FFFFFF",
"buttonBackgroundColor": "#FFC107",
"buttonTextColor": "#FFFFFF",
"primaryTextColor": "#212121",
"secondaryTextColor": "#757575"
}
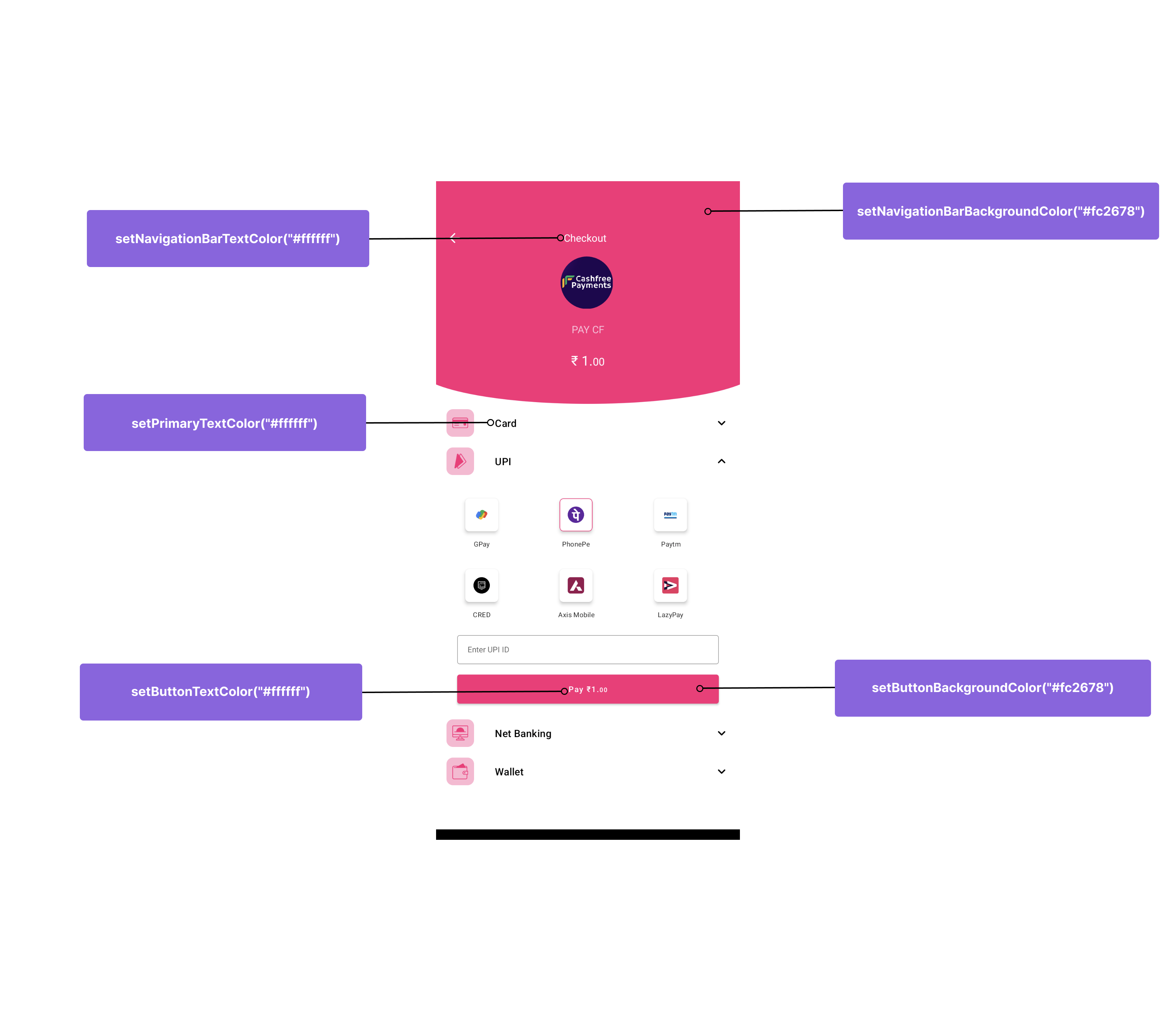
Theme options
Create a Drop Checkout Payment object
- Code Snippet to create a payment object for Drop Checkout (pre-built UI SDK)
let dropPaymentObject = {
"components": ["CARD", "UPI", "NB", "WALLET", "PAY_LATER", "EMI"],
"theme": {
"navigationBarBackgroundColor": "#E64A19",
"navigationBarTextColor": "#FFFFFF",
"buttonBackgroundColor": "#FFC107",
"buttonTextColor": "#FFFFFF",
"primaryTextColor": "#212121",
"secondaryTextColor": "#757575"
},
"session": {
"payment_session_id": "payment_session_id",
"orderID": "order_id",
"environment": "SANDBOX" //"SANDBOX" or "PRODUCTION"
}
}
If payment component object is not provided then all the payment modes that are enabled for the merchant account is shown.
Setup Payment Callback
The SDK exposes an interface CFCallback
to receive callbacks from the SDK once the payment flow ends.
This protocol comprises of 2 methods:
public void onVerify(result)
public void onError(error)
- Code snippet demonstrating it's usage:
function onDeviceReady() {
const callbacks = {
onVerify: function (result) {
let details = {
"orderID": result.orderID
}
console.log(details);
},
onError: function (error){
let errorObj = {
"orderID": result.orderID,
"status": error.status,
"code": error.code,
"type": error.type,
"message": error.message
}
console.log(errorObj);
}
}
CFPaymentGateway.setCallback(callbacks)
}
Sample Code
document.addEventListener('deviceready', onDeviceReady, false);
function onDeviceReady() {
console.log('Running cordova-' + cordova.platformId + '@' + cordova.version);
let dropElement = document.getElementById("onDrop");
dropElement.addEventListener("click", (e) => initiateDropPayment());
const callbacks = {
onVerify: function (result) {
let details = {
"orderID": result.orderID
}
console.log(details);
},
onError: function (error){
let errorObj = {
"orderID": result.orderID,
"status": error.status,
"code": error.code,
"type": error.type,
"message": error.message
}
console.log(errorObj);
}
}
CFPaymentGateway.setCallback(callbacks)
}
function initiateDropPayment() {
CFPaymentGateway.doDropPayment({
"components": ["CARD", "UPI", "NB", "WALLET", "PAY_LATER", "EMI"],
"theme": {
"navigationBarBackgroundColor": "#E64A19",
"navigationBarTextColor": "#FFFFFF",
"buttonBackgroundColor": "#FFC107",
"buttonTextColor": "#FFFFFF",
"primaryTextColor": "#212121",
"secondaryTextColor": "#757575"
},
"session": {
"payment_session_id": "payment_session_id",
"orderID": "order_id",
"environment": "SANDBOX" //"SANDBOX" or "PRODUCTION"
}
})
}
You can check our demo app located here.
Updated about 1 year ago