3.2 Initiate payment - UPI Checkout
UPI flow
UPI flow is the standard flow for Cashfree payment gateway's React Native SDK. In this flow, SDK provides a pre-built native screen to facilitate a quick integration with our payment gateway.
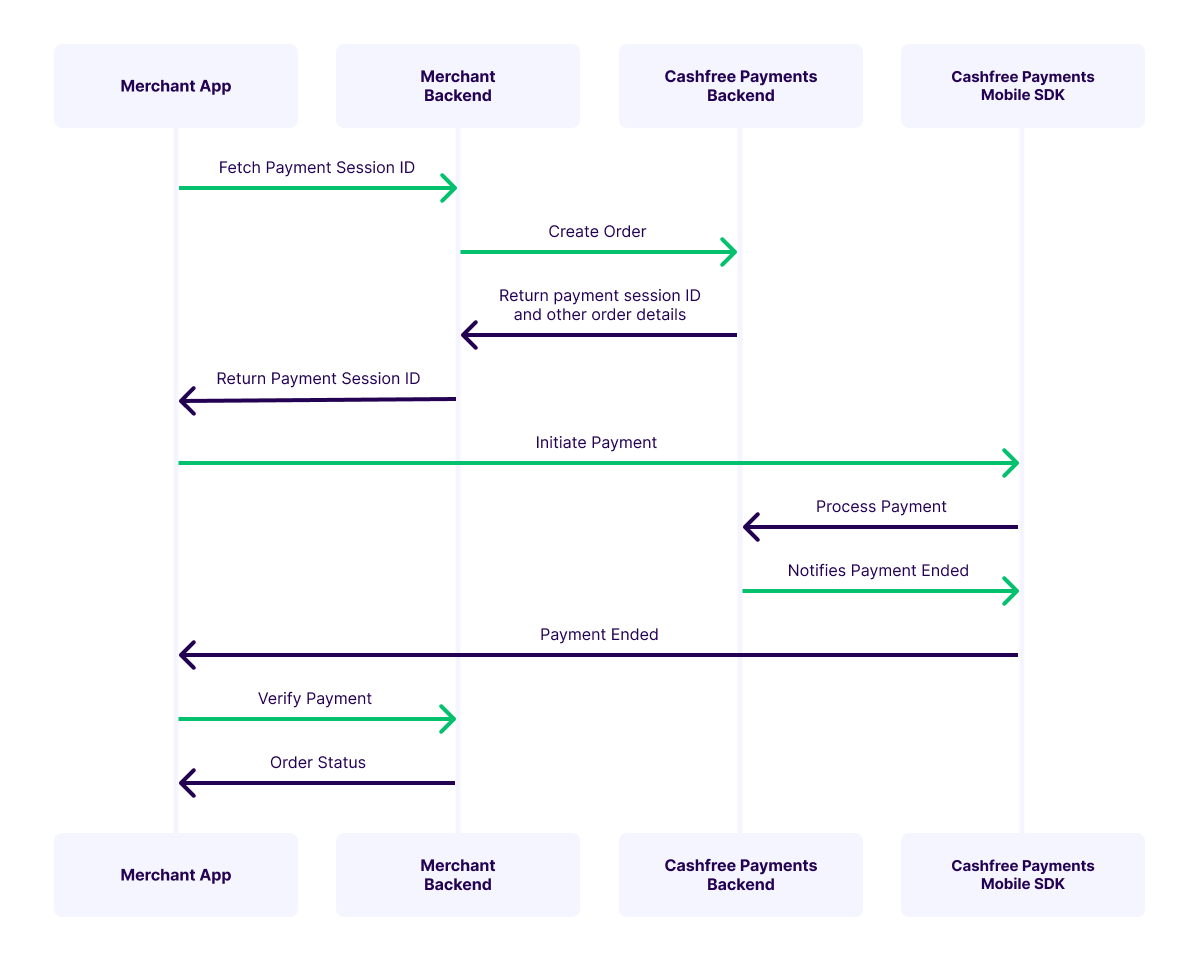
UPI Checkout
Initiating the payment
To initiate the UPI checkout payment in the SDK, follow these steps
- Create a CFSession object.
- Create a CFTheme object.
- Create a UPI Checkout Payment object.
- Set payment callback.
- Initiate the payment using the payment object created from [step 4]
Create a session
The payment_session_id
created from Step2 is used to authenticate the payment. The SDK exposes a class CFSession
class which accepts the payment_session_id, Environment and order ID values.
Cashfree provides two environments, one being the sandbox
environment for developers to test the payment flow and responses and the other being production
environment which gets shipped to production. This environment can be set in this session object.
The values for the environment can be either .SANDBOX
or .PRODUCTION
.
import {
CFEnvironment,
CFSession,
} from 'cashfree-pg-api-contract';
try {
const session = new CFSession(
'payment_session_id',
'order_id',
CFEnvironment.SANDBOX
);
} catch (e: any) {
console.log(e.message);
}
Set a Theme
const theme = new CFThemeBuilder()
.setNavigationBarBackgroundColor('#E64A19') // ios
.setNavigationBarTextColor('#FFFFFF') // ios
.setButtonBackgroundColor('#FFC107') // ios
.setButtonTextColor('#FFFFFF') // ios
.setPrimaryTextColor('#212121')
.setSecondaryTextColor('#757575') // ios
.build();
The CFThemeBuilder
class used to create CFTheme
used to set the theming for UPI checkout screen.
Create a UPI Checkout Payment object
- Code Snippet to create a payment object for UPI Checkout (pre-built UI SDK)
try {
const upiPayment = new CFUPIIntentCheckoutPayment(
session,
theme
);
CFPaymentGatewayService.doUPIPayment(upiPayment);
} catch (e: any) {
console.log(e.message);
}
If payment component object is not provided then all the payment modes that are enabled for the merchant account is shown.
Setup Payment Callback
The SDK exposes an interface CFCallback
to receive callbacks from the SDK once the payment flow ends.
This protocol comprises of 2 methods:
public void onVerify(String orderID)
public void onError(CFErrorResponse cfErrorResponse, String orderID)
- Code snippet demonstrating it's usage:
import {
CFErrorResponse,
CFPaymentGatewayService,
} from 'react-native-cashfree-pg-sdk';
export default class App extends Component {
constructor() {
super();
}
componentDidMount() {
console.log('MOUNTED');
CFPaymentGatewayService.setCallback({
onVerify(orderID: string): void {
this.changeResponseText('orderId is :' + orderID);
},
onError(error: CFErrorResponse, orderID: string): void {
this.changeResponseText(
'exception is : ' + JSON.stringify(error) + '\norderId is :' + orderID
);
},
});
}
componentWillUnmount() {
console.log('UNMOUNTED');
CFPaymentGatewayService.removeCallback();
}
}
Make sure to set the callback at componentDidMount and remove the callback at componentWillUnmount as this also handles the activity restart cases and prevents memory leaks.
Sample Code
import * as React from 'react';
import { Component } from 'react';
import {
CFErrorResponse,
CFPaymentGatewayService,
} from 'react-native-cashfree-pg-api';
import {
CFDropCheckoutPayment,
CFEnvironment,
CFPaymentComponentBuilder,
CFPaymentModes,
CFSession,
CFThemeBuilder,
} from 'cashfree-pg-api-contract';
export default class App extends Component {
constructor() {
super();
}
componentDidMount() {
console.log('MOUNTED');
CFPaymentGatewayService.setCallback({
onVerify(orderID: string): void {
this.changeResponseText('orderId is :' + orderID);
},
onError(error: CFErrorResponse, orderID: string): void {
this.changeResponseText(
'exception is : ' + JSON.stringify(error) + '\norderId is :' + orderID
);
},
});
}
componentWillUnmount() {
console.log('UNMOUNTED');
CFPaymentGatewayService.removeCallback();
}
async _startCheckout() {
try {
const session = new CFSession(
'payment_session_id',
'order_id',
CFEnvironment.SANDBOX
);
const theme = new CFThemeBuilder()
.setNavigationBarBackgroundColor('#E64A19')// ios
.setNavigationBarTextColor('#FFFFFF')// ios
.setButtonBackgroundColor('#FFC107')// ios
.setButtonTextColor('#FFFFFF')// ios
.setPrimaryTextColor('#212121')
.setSecondaryTextColor('#757575')// ios
.build();
const upiPayment = new CFUPIIntentCheckoutPayment(
session,
theme
);
CFPaymentGatewayService.doUPIPayment(upiPayment);
} catch (e: any) {
console.log(e.message);
}
}
}
You can check our demo app located here.
Resources
Cashfree UPI intent Simulator App
Subscribe to Developer Updates
Updated 22 days ago