Use this API to authenticate with the Cashfree system and obtain the authorization bearer token. All other API calls must have this token as Authorization header in the format 'Bearer ' (without quotes) for them to get processed. The generated token is valid for 600 seconds.
URLs
- Production/Live:https://payout-api.cashfree.com/payout/v1/authorize
- Sandbox/Test: https://payout-gamma.cashfree.com/payout/v1/authorize
Click to view the steps to generate a public key and the signature.
Public Key
If you do not have a static IP, you can generate a public key and pass it with the API request.
To generate a public key,
- Go Payouts Dashboard > Developers section on the left-side navigation > Payouts > Two-Factor Authentication > Public Key.
- Click Generate Public Key. The public key will be downloaded to your computer and the password to access it will be your email ID registered with Cashfree Payments. Only one Public Key can be generated at a time.
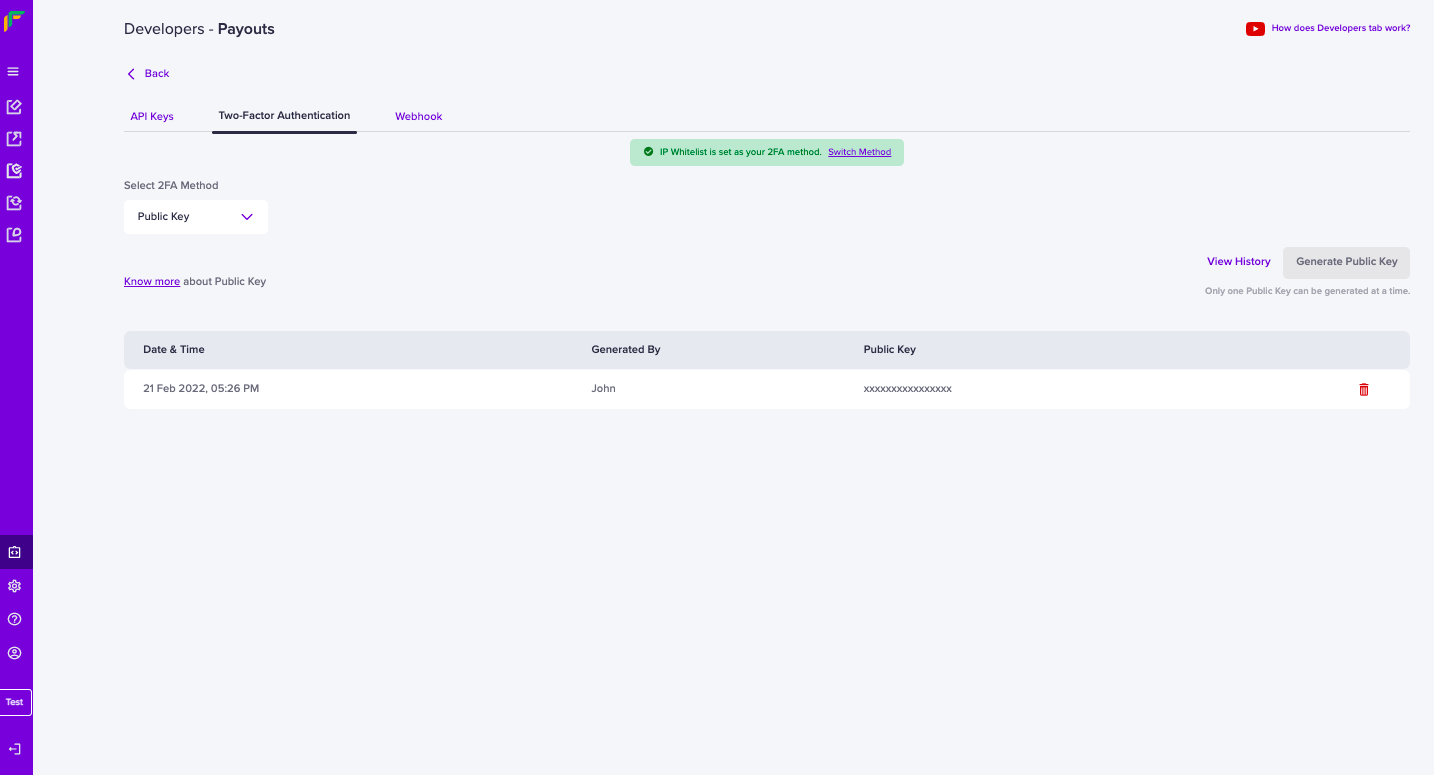
Public Key
Below are the steps to generate your signature:
- Retrieve your clientId (one which you are passing through the header X-Client-Id )
- Append this with CURRENT UNIX timestamp separated by a period (.)
- Encrypt this data using RSA encrypt with Public key you received – this is the signature.
- Pass this signature through the header X-Cf-Signature.
In the case of using our library, go through the libraries section. During the initialization process, you need to pass the key as a parameter.
<?php
public static function getSignature() {
$clientId = "<your clientId here>";
$publicKey =
openssl_pkey_get_public(file_get_contents("/path/to/certificate/public
_key.pem"));
$encodedData = $clientId.".".strtotime("now");
return static::encrypt_RSA($encodedData, $publicKey);
}
private static function encrypt_RSA($plainData, $publicKey) { if (openssl_public_encrypt($plainData, $encrypted, $publicKey,
OPENSSL_PKCS1_OAEP_PADDING))
$encryptedData = base64_encode($encrypted);
else return NULL;
return $encryptedData;
}
?>
private static String generateEncryptedSignature(String clientIdWithEpochTimestamp) {
// String clientIdWithEpochTimeStamp = clientId+"."+Instant.now().getEpochSecond();
String encrytedSignature = "";
try {
byte[] keyBytes = Files
.readAllBytes(new File("/Users/sameera/Downloads/payout_test_public_key.pem").toPath()); // Absolute Path to be replaced
String publicKeyContent = new String(keyBytes);
System.out.println(publicKeyContent);
publicKeyContent = publicKeyContent.replaceAll("[\\t\\n\\r]", "")
.replace("-----BEGIN PUBLIC KEY-----", "").replace("-----END PUBLIC KEY-----", "");
KeyFactory kf = KeyFactory.getInstance("RSA");
System.out.println(publicKeyContent);
X509EncodedKeySpec keySpecX509 = new X509EncodedKeySpec(
Base64.getDecoder().decode(publicKeyContent));
RSAPublicKey pubKey = (RSAPublicKey) kf.generatePublic(keySpecX509);
final Cipher cipher = Cipher.getInstance("RSA/ECB/OAEPWithSHA-1AndMGF1Padding");
cipher.init(Cipher.ENCRYPT_MODE, pubKey);
encrytedSignature = Base64.getEncoder().encodeToString(cipher.doFinal(clientIdWithEpochTimestamp.getBytes()));
System.out.println(encrytedSignature);
} catch (Exception e) {
e.printStackTrace();
}
return encrytedSignature;
}
from cashfree_sdk.payouts import Payouts
// Initialise the SDK, pass public key for dynamic IP
Payouts.init("<client_id>", "<client_secret>", "PROD", public_key= b'public key')
//require CashfreeSDK
const cfSdk = require('cashfree-sdk');
//access the PayoutsSdk from CashfreeSDK
const {Payouts} = cfSdk;
// Instantiate Cashfree Payouts
const payoutsInstance = new Payouts({
env: 'TEST',
clientId: '<CLIENT_ID>',
clientSecret: '<CLIENT_SECRET>',
pathToPublicKey: '/path/to/your/public/key/file.pem',
//"publicKey": "ALTERNATIVE TO SPECIFYING PATH (DIRECTLY PASTE PublicKey)"
});